diff --git a/proposals/0000-reduce-fragmentation.md b/proposals/0000-reduce-fragmentation.md
new file mode 100644
index 00000000..40609598
--- /dev/null
+++ b/proposals/0000-reduce-fragmentation.md
@@ -0,0 +1,1153 @@
+---
+title: React DOM for Native
+author:
+- Nicolas Gallagher
+date: 2022-08-03
+updated: 2023-01-31
+---
+
+# RFC: React DOM for Native
+
+## Summary
+
+This is a proposal to incrementally reduce the API fragmentation faced by developers using React to target multiple platforms via code shared between native and web. The proposed cross-platform user interface APIs are a subset of existing web standards for DOM, CSS, and HTML - "Strict DOM". The proposed changes are overwhelmingly additive and do not require migration of existing React Native UI code (deprecations are optional / follow up work). Incremental progress can help to reduce the fragmentation between React Native and React DOM components, while the ability to run React DOM code (with minor modifications) on native is a longer-term goal.
+
+## Motivation
+
+React Native currently includes many APIs that are modelled on Web APIs but do not conform to the standards of those Web APIs. React Native also includes many APIs that achieve the same results on Android and iOS but are exposed as 2 different props. And React Native includes several APIs that have known performance (network and runtime) drawbacks on Web.
+
+This proposal aims to allow developers to target more platforms with cross-platform APIs, and deliver better performance when targeting browsers. Features for Android, iOS, and Web are unified by aligning with the Web standard. Supporting standards helps to:
+
+* minimize the overhead when running in browsers;
+* reduce developer education required to learn features;
+* set clear and cohesive API end-state expectations for contributors to aim for;
+* accelerate the framework's development by avoiding API design costs;
+* support backwards compatibility;
+* develop universal codebases.
+
+The existing solution for targeting web with React Native is to use React Native for Web. React Native for Web is a user-space library built on top of React DOM and native DOM APIs. It shims React Native components and APIs on the web. The tooling for an existing React DOM app simply maps the 'react-native' export to 'react-native-web'.
+
+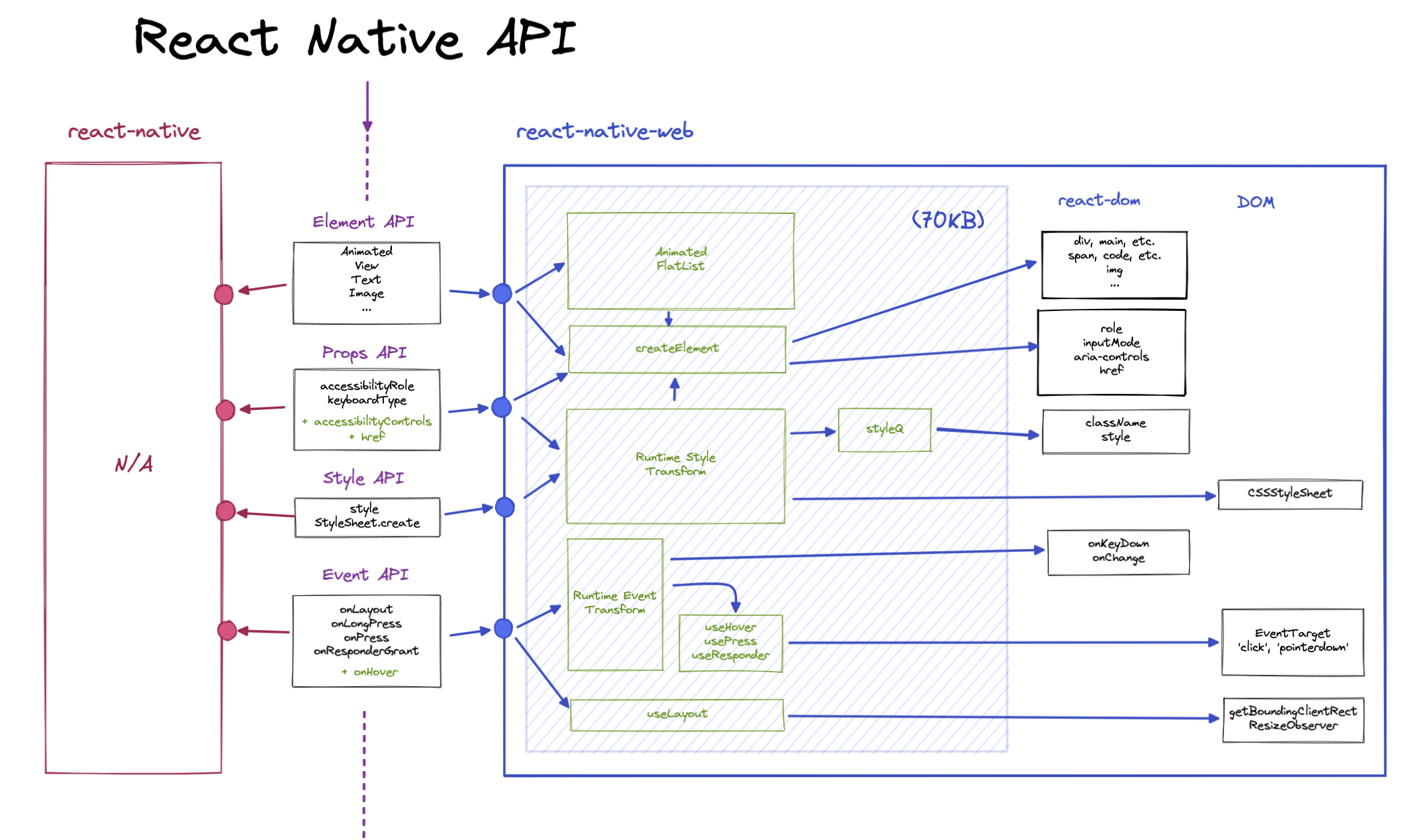
+
+This is the most complete and widely used shim, but comes with considerable DX and UX costs on the web. The shim must implement a large surface area of fragmented APIs, and it needs to modify standard APIs and objects (e.g., events) to match React Native's non-standard implementations.
+
+In contrast, implementing a "Strict DOM" subset in React Native shifts the weight of bridging native and web apps onto React Native, where it can be done most efficiently. Although React Native will not support all the features available on web, it will still support a greater expanded feature set relative to React Native today. On the web, we would only need to combine React DOM with a white-label CSS compiler like stylex.
+
+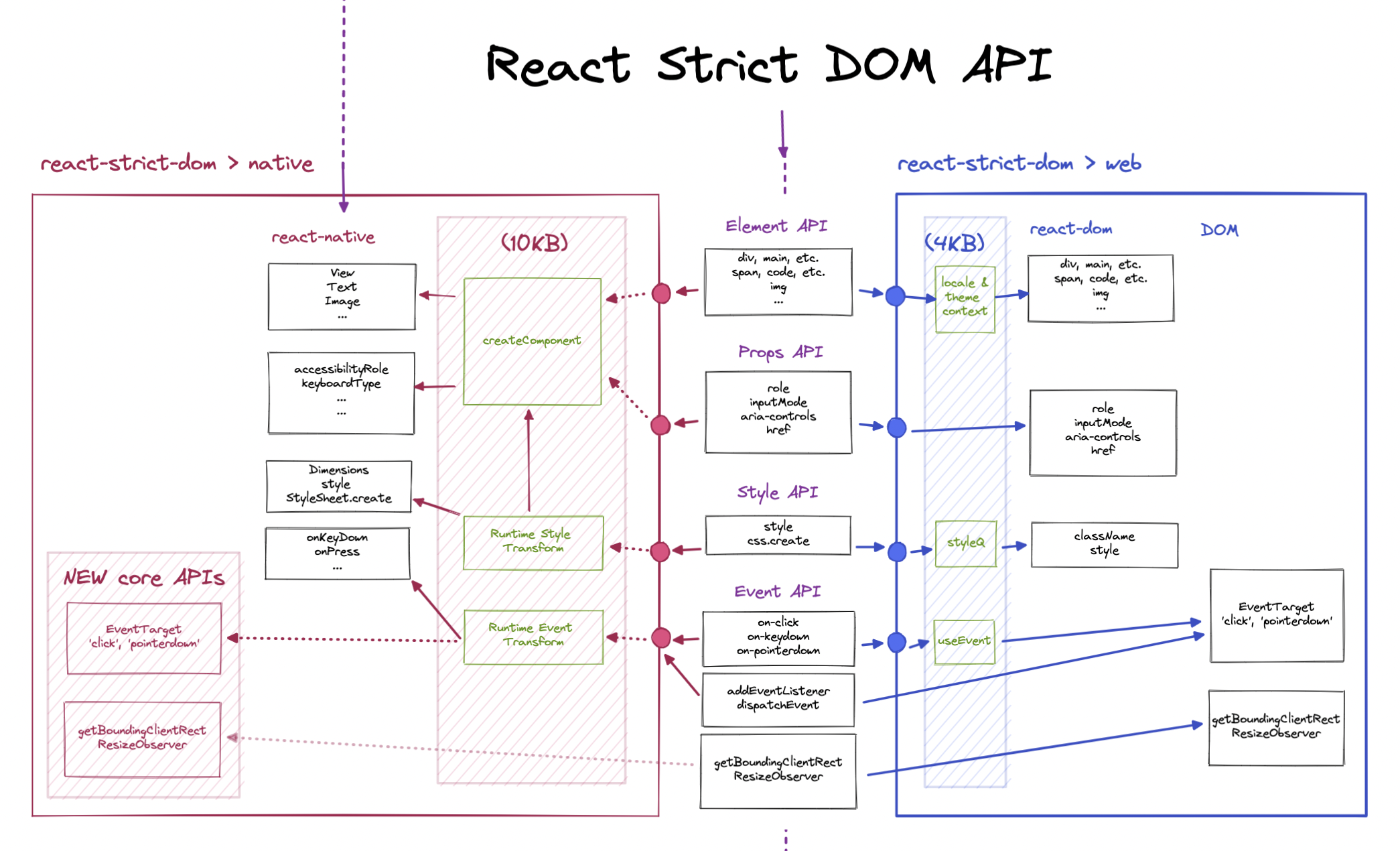
+
+## Detailed design
+
+The "complete" API involved is broken down into the following sections.
+
+* [Environment API](#environment-api). Global APIs to add to the host environment.
+* [Elements API](#elements-api-dom-subset). DOM APIs to add to host component instances.
+* [Components API](#components-api-dom-subset). React DOM components to add to React Native.
+* [Props API](#props-api-react-dom-subset). React DOM component props to add to new components, and existing React Native components as part of an incremental strategy.
+* [Styling API](#styles-api-css-subset). CSS APIs to add to the React Native environment.
+
+A simplified example of the user-space code we'd aim to support is as follows:
+
+```js
+import { html, css } from 'react-native/dom';
+
+export function VStackPanel(props) {
+ const connectedCallback = function (node) {
+ const handler = () => {
+ const { offsetWidth } = e.target;
+ // ...
+ };
+ const options = { capture: true };
+ node.addEventListener('pointerdown', handler, options);
+ return function disconnected() {
+ node.removeEventListener('pointerdown', handler, options)
+ }
+ };
+
+ return (
+ // Use HTML element
+
+ )
+}
+```
+
+But there are many incremental steps that can be taken along the way.
+
+## Implementation and adoption strategy
+
+The proposal is to support both an incremental adoption strategy, as well as a separate "React DOM" bindings exports. The incremental strategy would find ways to align existing APIs in React Native with their React DOM equivalents, and gradually deprecate the non-standard APIs. Whereas the separate bindings export would be designed to provide most of what is needed for a direct compatibility layer with React DOM.
+
+This would ensure a significant amount of overlap between the existing React Native components and the dedicated "React DOM" compatibility API, allowing for co-mingling of code and reduced developer education. There is no suggestion of deprecating the current React Native components and APIs at this stage.
+
+### Reduce API fragmentation across platforms
+
+The initial step is to incrementally reduce existing API fragmenetation across platforms. This involves consolidating the different props, styles, and events for similar functionality across different platforms. The APIs that we consolidate upon are the W3C standards, as React Native is already fairly closely aligned with these standards.
+
+Doing this will make it easier for web developers to work with React Native's existing components and related APIs. This is the path for developers to gradually migrate their existing React Native code into a form that is more aligned with the web. And it will allow React Native for Web to be smaller and faster, providing better results on web via the existing tools that developers use today.
+
+One example of this kind of incremental change is to map props in the existing core components (i.e., `View`, `Text`, etc.) to the W3C equivalents: `id` to `nativeID`, `aria-label` to `accessibilityLabel`, and so on. React Native for Web currently "owns" this translation step, but we would instead move it into React Native to claw back performance on web. For developers familiar with React DOM, there will be less of a learning curve to applying that knowledge to React Native.
+
+```jsx
+
+```
+
+```jsx
+
+```
+
+```jsx
+
+
+
+```
+
+Example of how to map `srcSet` to `source`:
+
+```js
+const { crossOrigin, referrerPolicy, srcSet } = props;
+const source = [];
+const srcList = srcSet.split(', ');
+srcList.forEach((src) => {
+ const [uri, xscale] = src.split(' ');
+ const scale = parseInt(xscale.split('x')[0], 10);
+ const headers = {};
+ if (crossOrigin === 'use-credentials') {
+ headers['Access-Control-Allow-Credentials'] = true;
+ }
+ if (referrerPolicy != null) {
+ headers['Referrer-Policy'] = referrerPolicy;
+ }
+ source.push({ headers, height, scale, uri, width });
+});
+```
+
+Example of how to map `autoComplete` to Android's `autoComplete` and iOS's `textContentType`:
+
+```js
+// https://reactnative.dev/docs/textinput#autocomplete-android
+const autoCompleteAndroid = {
+ 'address-line1': 'postal-address-region',
+ 'address-line2': 'postal-address-locality',
+ bday: 'birthdate-full',
+ 'bday-day': 'birthdate-day',
+ 'bday-month': 'birthdate-month',
+ 'bday-year': 'birthdate-year',
+ 'cc-csc': 'cc-csc',
+ 'cc-exp': 'cc-exp',
+ 'cc-exp-month': 'cc-exp-month',
+ 'cc-exp-year': 'cc-exp-year',
+ 'cc-number': 'cc-number',
+ country: 'postal-address-country',
+ 'current-password': 'password',
+ email: 'email',
+ name: 'name',
+ 'additional-name': 'name-middle',
+ 'family-name': 'name-family',
+ 'given-name': 'name-given',
+ 'honorific-prefix': 'namePrefix',
+ 'honorific-suffix': 'nameSuffix',
+ 'new-password': 'password-new',
+ off: 'off',
+ 'one-time-code': 'sms-otp',
+ 'postal-code': 'postal-code',
+ sex: 'gender',
+ 'street-address': 'street-address',
+ tel: 'tel',
+ 'tel-country-code': 'tel-country-code',
+ 'tel-national': 'tel-national',
+ username: 'username'
+};
+
+// https://reactnative.dev/docs/textinput#textcontenttype-ios
+const autoCompleteIOS = {
+ 'address-line1': 'streetAddressLine1',
+ 'address-line2': 'streetAddressLine2',
+ 'cc-number': 'creditCardNumber',
+ 'current-password': 'password',
+ country: 'countryName',
+ email: 'emailAddress',
+ name: 'name',
+ 'additional-name': 'middleName',
+ 'family-name': 'familyName',
+ 'given-name': 'givenName',
+ nickname: 'nickname',
+ 'honorific-prefix': 'name-prefix',
+ 'honorific-suffix': 'name-suffix',
+ 'new-password': 'newPassword',
+ off: 'none',
+ 'one-time-code': 'oneTimeCode',
+ organization: 'organizationName',
+ 'organization-title': 'jobTitle',
+ 'postal-code': 'postalCode',
+ 'street-address': 'fullStreetAddress',
+ tel: 'telephoneNumber',
+ url: 'URL',
+ username: 'username'
+};
+```
+
+Where possible we should make these changes to the native code. However, we will prioritize user-space shims in JavaScript where they are faster to ship and allow us to gather feedback/support for this overall direction.
+
+Beyond props, there is also the opportunity to reduce fragementation in styling APIs.
+
+* [ ] Don't hard crash on unknown or invalid style properties and values.
+* [ ] Deprecate `StyleSheet.absoluteFill`. Use `position:'absolute';inset:0;` instead.
+* [ ] Deprecate `StyleSheet.absoluteFillObject`.
+* [ ] Deprecate `StyleSheet.compose()`. Use existing `[ a, b ]` syntax instead.
+* [ ] Deprecate `StyleSheet.flatten()`. This API encourages runtime introspection of styles in render calls. This pattern is a performance cost (flattening arrays of objects), and prevents us from supporting build-time optimizations for web (e.g., extracting styles to CSS files).
+* [ ] Deprecate or rename `setStyleAttributePreprocessor` (e.g., `unstable_setStyleAttributePreprocessor`).
+* [ ] `StyleSheet.create()` should obfuscate styles to prevent introspection (i.e., revert identify function change). On web, we need to be able to remove the JavaScript style objects from bundles to support build-time optimization like extraction to CSS files.
+
+To encourage React Native library and product developers to proactively migrate to these new APIs, React Native for Web plans to only support these W3C standards-based APIs in future versions. This will allow us to incrementally add APIs to React Native without needing to commit to simultaneously deprecating APIs and migrating existing React Native code. Existing React Native developers must adopt these APIs if they wish to support web.
+
+### Create a separate package or export for DOM bindings
+
+The next step would be to begin exporting separate React DOM bindings from React Native. This would aim to allow code originally written with React DOM to run on React Native with only minor modifications. There would be no need to use React Native for Web, and the web layer in this scenario would be a lot smaller, limited mostly to implementing the modern W3C events API and integrating a style API that allows for static extraction to optimized CSS.
+
+Even this can be done in incremental fashion, with early (user-space) prototypes to get feedback. Most tags can be mapped to existing React Native components and props, in the reverse of the [mapping used by React Native for Web](https://github.com/necolas/react-native-web/blob/b2eb3ca03ffc4b8570591b6c2082c6e4edae0e7f/packages/react-native-web/src/modules/AccessibilityUtil/propsToAccessibilityComponent.js#L12-L30).
+
+### Equivalent API for Web
+
+In parallel, a user-space layer over React DOM would ensure equivalent APIs between native and web, by converging them both towards a desired the "end state" where the differences between React Native and React DOM are erased while landing on a "modern" flavor of React DOM.
+
+The benefit of initially doing this in user-space is that it reduces the burden of complex migratations for React Native and React DOM (e.g., the changes to React DOM event types, the `style` prop, and explicit imports of component types), while allowing us to validate and iterate on the proposed end-state APIs with interested parties. This is currently blocked on OSS-ing of stylex.
+
+## How we teach this
+
+Teaching these APIs is simplified by developer familiarity with existing DOM / React DOM APIs.
+
+## Drawbacks
+
+Many of these features can be shimmed in user-space, at the cost of runtime overhead for native or web platforms. The runtime overhead for web is untenable. The runtime overhead of shifting shims into the React Native JS has yet to be established.
+
+This adds redundancy to the React Native API, as the proposal is to make additive changes and avoid requiring removal of existing APIs in the short term.
+
+We have to be very intentional about how much compatibility with open web standards we can promise. Some cross-platform limitations are rooted in significant differences in the host platforms APIs (e.g., accessibility) and the lack of 99% compat may frustrate users.
+
+Aiming for a degree of React DOM compatibility shifts the burden of missing features onto React Native. Developers may not be used to an API that is complete on web but partially implemented on native platforms.
+
+-----
+
+# Environment APIs
+
+## `window`
+
+### Events
+
+* [ ] Receives all W3C events dispatched to components (capture and bubble phase). Resolves proposal [#249](https://github.com/react-native-community/discussions-and-proposals/issues/249).
+* [ ] Dispatches [`resize`](https://developer.mozilla.org/en-US/docs/Web/API/Window/resize_event).
+
+### Properties
+
+* [ ] [`window.devicePixelRatio`](https://developer.mozilla.org/en-US/docs/Web/API/Window/devicePixelRatio)
+* [ ] [`window.navigator`](https://developer.mozilla.org/en-US/docs/Web/API/Navigator)
+ * [ ] [`clipboard`](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/clipboard)
+ * [ ] [`languages`](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/languages)
+ * [ ] [`permissions`](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/permissions)
+ * [ ] [`vibrate()`](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/vibrate)
+* [ ] [`window.performance`](https://developer.mozilla.org/en-US/docs/Web/API/performance_property)
+
+### Methods
+
+* [ ] `window.addEventListener()`
+* [ ] `window.dispatchEvent()`
+* [ ] [`window.getSelection()`](https://developer.mozilla.org/en-US/docs/Web/API/Window/getSelection)
+* [ ] `window.removeEventListener()`
+
+### APIs
+
+* [ ] [`window.fetch`](https://developer.mozilla.org/en-US/docs/Web/API/fetch)
+* [ ] [`window.matchMedia`](https://developer.mozilla.org/en-US/docs/Web/API/Window/matchMedia). Resolves proposal [#350](https://github.com/react-native-community/discussions-and-proposals/issues/350).
+* [ ] [`IntersectionObserver`](https://developer.mozilla.org/en-US/docs/Web/API/IntersectionObserver) API for observing the intersection of target elements. This can also be used as a building block for performance tooling.
+* [ ] [`MutationObserver`](https://developer.mozilla.org/en-US/docs/Web/API/MutationObserver) API for watching changes to the host node tree. This can also be used as a building block for performance tooling.
+* [ ] [`ResizeObserver`](https://developer.mozilla.org/en-US/docs/Web/API/ResizeObserver) API for responding the changes in the size of elements. Note that this API no longer includes positional coordinates and is optimized for width/height information.
+
+## `document`
+
+Support the `document` object for common patterns such as listening to visibility changes, and listening to capture or bubble phase events for the entire application (e.g., "outside click" pattern), etc.
+
+The `document` object might not be exposed as a global, and would instead be accessed via `node.getRootNode()` to ensure code accounts for multi-window / multi-root scenarios that are more common for React Native apps than React DOM apps (rendering into sourceless iframes is a similar use case).
+
+### Events
+
+* [ ] [`scroll`](https://developer.mozilla.org/en-US/docs/Web/API/Document/scroll_event).
+* [ ] [`visibilitychange`](https://developer.mozilla.org/en-US/docs/Web/API/Document/visibilitychange_event). Can replace the `AppState` API.
+
+### Properties
+
+- [ ] [`document.activeElement`](https://developer.mozilla.org/en-US/docs/Web/API/Document/activeElement).
+- [ ] [`document.defaultView`](https://developer.mozilla.org/en-US/docs/Web/API/Document/defaultView). Returns the `window` object associated with a `document`, or `null` if none is available.
+- [ ] [`document.visibilityState`](https://developer.mozilla.org/en-US/docs/Web/API/Document/visibilityState)
+
+### Methods
+
+- [ ] [`document.getElementFromPoint(x,y)`](https://developer.mozilla.org/en-US/docs/Web/API/Document/elementFromPoint). Resolves proposal [#501](https://github.com/react-native-community/discussions-and-proposals/issues/501).
+
+-----
+
+# Elements API (DOM subset)
+
+Subset of cross-platform DOM APIs exposed on element instances. This would be an imperative API for working with the Shadow tree in React Native. Based on data from [MDN Web API](https://developer.mozilla.org/en-US/docs/Web/API), please refer to the MDN links for complete DOM APIs and comment if you believe any should be included in the proposed subset.
+
+In practice, this DOM API subset is generally limited to the “best practice” APIs that can safely be used with React DOM (i.e., read-only operations that don’t modify the DOM tree), and most React components should already be limited to this subset. One of the important details of this API are how it supports the different ways of [determining the dimensions of elements](https://developer.mozilla.org/en-US/docs/Web/API/CSS_Object_Model/Determining_the_dimensions_of_elements).
+
+## EventTarget
+
+The `EventTarget` API is implemented in JS environments, and should be available on React Native host elements. This feature is commonly used on web, and could be used by React Native developers to support more complex features without first requiring further core API changes to support each use case.
+
+### Methods
+
+- [ ] [`EventTarget.addEventListener()`](https://developer.mozilla.org/en-US/docs/Web/API/EventTarget/addEventListener). Registers an event handler to a specific event type on the element.
+- [ ] [`EventTarget.dispatchEvent()`](https://developer.mozilla.org/en-US/docs/Web/API/EventTarget/dispatchEvent). Dispatches an event to this node in the DOM and returns a boolean value that indicates whether no handler canceled the event.
+- [ ] [`EventTarget.removeEventListener()`](https://developer.mozilla.org/en-US/docs/Web/API/EventTarget/removeEventListener). Removes an event listener from the element.
+
+## Event and CustomEvent
+
+[Event()](https://developer.mozilla.org/en-US/docs/Web/API/Event/Event) and [CustomEvent()](https://developer.mozilla.org/en-US/docs/Web/API/CustomEvent) represent events initialized by developers and that can be dispatched to elements using `EventTarget.dispatchEvent()`.
+
+## Node
+
+The DOM Node interface is an abstract base class upon which many other DOM API objects are based, thus letting those object types to be used similarly and often interchangeably. All objects that implement Node functionality are based on one of its subclasses. Every kind of host node is represented by an interface based on Node.
+
+In some cases, a particular feature of the base Node interface may not apply to one of its child interfaces; in that case, the inheriting node may return null or throw an exception, depending on circumstances.
+
+### Static properties
+
+[Node type constants](https://developer.mozilla.org/en-US/docs/Web/API/Node/nodeType#value)
+
+- [x] `Node.ELEMENT_NODE` (1)
+- [x] `Node.TEXT_NODE` (3)
+- [x] `Node.DOCUMENT_NODE` (9)
+
+[Document position constants](https://developer.mozilla.org/en-US/docs/Web/API/Node/compareDocumentPosition#value)
+
+- [x] `Node.DOCUMENT_POSITION_DISCONNECTED` (1)
+- [x] `Node.DOCUMENT_POSITION_PRECEDING` (2)
+- [x] `Node.DOCUMENT_POSITION_FOLLOWING` (4)
+- [x] `Node.DOCUMENT_POSITION_CONTAINS` (8)
+- [x] `Node.DOCUMENT_POSITION_CONTAINED_BY` (16)
+
+### Instance properties
+
+- [x] [`node.childNodes`](https://developer.mozilla.org/en-US/docs/Web/API/Node/childNodes). Not a live collection.
+- [x] [`node.firstChild`](https://developer.mozilla.org/en-US/docs/Web/API/Node/firstChild)
+- [x] [`node.isConnected`](https://developer.mozilla.org/en-US/docs/Web/API/Node/isConnected)
+- [x] [`node.lastChild`](https://developer.mozilla.org/en-US/docs/Web/API/Node/lastChild)
+- [x] [`node.nextSibling`](https://developer.mozilla.org/en-US/docs/Web/API/Node/nextSibling)
+- [x] [`node.nodeName`](https://developer.mozilla.org/en-US/docs/Web/API/Node/nodeName)
+- [x] [`node.nodeType`](https://developer.mozilla.org/en-US/docs/Web/API/Node/nodeType)
+- [x] [`node.nodeValue`](https://developer.mozilla.org/en-US/docs/Web/API/Node/nodeValue) (always null)
+- [x] [`node.parentElement`](https://developer.mozilla.org/en-US/docs/Web/API/Node/parentElement)
+- [x] [`node.parentNode`](https://developer.mozilla.org/en-US/docs/Web/API/Node/parentNode)
+- [x] [`node.previousSibling`](https://developer.mozilla.org/en-US/docs/Web/API/Node/previousSibling)
+- [x] [`node.textContent`](https://developer.mozilla.org/en-US/docs/Web/API/Node/textContent)
+
+### Instances methods
+
+Node inherits methods from its parent, EventTarget.
+
+- [x] [`node.compareDocumentPosition()`](https://developer.mozilla.org/en-US/docs/Web/API/Node/compareDocumentPosition)
+- [x] [`node.contains()`](https://developer.mozilla.org/en-US/docs/Web/API/Node/contains). Returns true or false value indicating whether or not a node is a descendant of the calling node.
+- [ ] [`node.getRootNode()`](https://developer.mozilla.org/en-US/docs/Web/API/Node/getRootNode). Returns the context object's root which optionally includes the shadow root if it is available..
+- [x] [`node.hasChildNodes()`](https://developer.mozilla.org/en-US/docs/Web/API/Node/hasChildNodes).
+
+## Element
+
+Element is the most general base class from which all element objects (i.e. objects that represent elements) in a Document inherit. It only has methods and properties common to all kinds of elements. More specific classes inherit from Element.
+
+### Instance properties
+
+Element inherits properties from its parent interface, Node, and by extension that interface's parent, EventTarget.
+
+- [x] [`element.childElementCount`](https://developer.mozilla.org/en-US/docs/Web/API/Element/childElementCount).
+- [x] [`element.children`](https://developer.mozilla.org/en-US/docs/Web/API/Element/children). Not a live collection.
+- [x] [`element.clientHeight`](https://developer.mozilla.org/en-US/docs/Web/API/Element/clientHeight) [Read only]. Returns a number representing the inner height of the element.
+- [x] [`element.clientLeft`](https://developer.mozilla.org/en-US/docs/Web/API/Element/clientLeft) [Read only]. Returns a number representing the width of the left border of the element.
+- [x] [`element.clientTop`](https://developer.mozilla.org/en-US/docs/Web/API/Element/clientTop) [Read only]. Returns a number representing the width of the top border of the element.
+- [x] [`element.clientWidth`](https://developer.mozilla.org/en-US/docs/Web/API/Element/clientWidth) [Read only]. Returns a number representing the inner width of the element.
+- [x] [`element.firstElementChild`](https://developer.mozilla.org/en-US/docs/Web/API/Element/firstElementChild).
+- [x] [`element.id`](https://developer.mozilla.org/en-US/docs/Web/API/Element/id) [Read only]. Is a DOMString representing the id of the element.
+- [x] [`element.lastElementChild`](https://developer.mozilla.org/en-US/docs/Web/API/Element/lastElementChild).
+- [x] [`element.nextElementSibling`](https://developer.mozilla.org/en-US/docs/Web/API/Element/nextElementSibling).
+- [x] [`element.previousElementSibling`](https://developer.mozilla.org/en-US/docs/Web/API/Element/previousElementSibling).
+- [x] [`element.scrollHeight`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollHeight) [Read only]. Returns a number representing the scroll view height of an element.
+- [x] [`element.scrollLeft`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollLeft). Is a number representing the left scroll offset of the element.
+- [x] [`element.scrollTop`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollTop). A number representing number of pixels the top of the element is scrolled vertically.
+- [x] [`element.scrollWidth`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollWidth) [Read only]. Returns a number representing the scroll view width of the element.
+- [x] [`element.tagName`](https://developer.mozilla.org/en-US/docs/Web/API/Element/tagName) (alias for nodeName).
+
+### Instance methods
+
+Element inherits methods from its parents Node, and its own parent, EventTarget.
+
+- [ ] [`element.computedStyleMap()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/computedStyleMap). Returns a StylePropertyMapReadOnly interface which provides a read-only representation of a CSS declaration block that is an alternative to CSSStyleDeclaration.
+- [ ] [`element.getAttribute()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/getAttribute). Returns the value of a specified attribute on the element.
+- [x] [`element.getBoundingClientRect()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/getBoundingClientRect). Returns the size of an element and its position relative to the viewport.
+- [ ] [`element.getClientRects()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/getClientRects). Returns a collection of `DOMRect` objects that indicate the bounding rectangles for each CSS border box in a client.
+- [ ] [`element.hasAttribute()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/hasAttribute). Returns a Boolean value indicating whether the specified element has the specified attribute or not.
+- [ ] [`element.hasPointerCapture()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/hasPointerCapture). Checks whether the element on which it is invoked has pointer capture for the pointer identified by the given pointer ID.
+- [ ] [`element.scroll()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scroll). Scrolls to a particular set of coordinates inside a given element. (Note that this would be async in React Native.)
+- [ ] [`element.scrollBy()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollBy). Scrolls an element by the given amount. (Note that this would be async in React Native.)
+- [ ] [`element.scrollIntoView()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollIntoView). Scrolls the page until the element gets into the view. (Note that this would be async in React Native.)
+- [ ] [`element.scrollTo()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollTo). Alias for `scroll()`.
+- [ ] [`element.setPointerCapture()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/setPointerCapture). Used to designate a specific element as the capture target of future pointer events. Subsequent events for the pointer will be targeted at the capture element until capture is released.
+- [ ] [`element.releasePointerCapture()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/releasePointerCapture). Releases (stops) pointer capture that was previously set for a specific (PointerEvent) pointer.
+
+### Events
+
+Listen to these events using `addEventListener()` or by assigning an event handler to the equivalent component prop.
+
+- [ ] `error`. Fired when a resource failed to load, or can't be used. For example, if a script has an execution error or an image can't be found or is invalid. Also available via the `on-error` property.
+- [ ] `scroll`. Fired when the document view or an element has been scrolled. Also available via the `on-scroll` property.
+- [ ] `select`. Fired when some text has been selected. Also available via the `on-select` property.
+- [ ] `wheel`. Fired when the user rotates a wheel button on a pointing device (typically a mouse). Also available via the `on-wheel` property.
+
+Clipboard events
+
+- [ ] [`copy`](https://developer.mozilla.org/en-US/docs/Web/API/Element/copy_event). Fired when the user initiates a copy action through the browser's user interface. Also available via the `on-copy` property.
+- [ ] [`cut`](https://developer.mozilla.org/en-US/docs/Web/API/Element/cut_event). Fired when the user initiates a cut action through the browser's user interface. Also available via the `on-cut` prop.
+- [ ] [`paste`](https://developer.mozilla.org/en-US/docs/Web/API/Element/paste_event). Fired when the user initiates a paste action through the browser's user interface. Also available via the `on-paste` prop.
+
+Focus events
+
+- [ ] [`blur`](https://developer.mozilla.org/en-US/docs/Web/API/Element/blur_event). Fired when an element has lost focus. Also available via the `on-blur` prop.
+- [ ] [`focus`](https://developer.mozilla.org/en-US/docs/Web/API/Element/focus_event). Fired when an element has gained focus. Also available via the `on-focus` prop.
+- [ ] [`focusin`](https://developer.mozilla.org/en-US/docs/Web/API/Element/focusin_event). Fired when an element is about to gain focus.
+- [ ] [`focusout`](https://developer.mozilla.org/en-US/docs/Web/API/Element/focusout_event). Fired when an element is about to lose focus.
+
+Keyboard events
+
+- [ ] [`keydown`](https://developer.mozilla.org/en-US/docs/Web/API/Element/keydown_event). Fired when a key is pressed. Also available via the `on-keydown` prop.
+- [ ] [`keyup`](https://developer.mozilla.org/en-US/docs/Web/API/Element/keyup_event). Fired when a key is released. Also available via the `on-keyup` prop.
+
+Pointer events
+
+- [ ] [`auxclick`](https://www.w3.org/TR/pointerevents3/#the-click-auxclick-and-contextmenu-events). Fired when a non-primary pointing device button (e.g., any mouse button other than the left button) has been pressed and released on an element. Also available via the `onAuxClick` prop.
+- [ ] [`click`](https://www.w3.org/TR/pointerevents3/#the-click-auxclick-and-contextmenu-events). Fired when a pointing device button (e.g., a mouse's primary button) is pressed and released on a single element. Also available via the `onClick` prop.
+- [ ] [`contextmenu`](https://www.w3.org/TR/pointerevents3/#the-click-auxclick-and-contextmenu-events). Fired when the user attempts to open a context menu. Also available via the oncontextmenu property.
+- [ ] [`gotpointercapture`](https://www.w3.org/TR/pointerevents3/#the-gotpointercapture-event). Fired when an element captures a pointer using `setPointerCapture()`. Also available via the `on-gotpointercapture` prop.
+- [ ] [`lostpointercapture`](https://www.w3.org/TR/pointerevents3/#the-lostpointercapture-event). Fired when a captured pointer is released. Also available via the `on-lostpointercapture` prop.
+- [ ] [`pointercancel`](https://www.w3.org/TR/pointerevents3/#the-pointercancel-event). Fired when a pointer event is canceled. Also available via the `on-pointercancel` prop.
+- [ ] [`pointerdown`](https://www.w3.org/TR/pointerevents3/#the-pointerdown-event). Fired when a pointer becomes active. Also available via the `on-pointerdown` prop.
+- [ ] [`pointerenter`](https://www.w3.org/TR/pointerevents3/#the-pointerenter-event). Fired when a pointer is moved into the hit test boundaries of an element or one of its descendants. Also available via the `on-pointerenter` prop.
+- [ ] [`pointerleave`](https://www.w3.org/TR/pointerevents3/#the-pointerleave-event). Fired when a pointer is moved out of the hit test boundaries of an element. Also available via the `on-pointerleave` prop.
+- [ ] [`pointermove`](https://www.w3.org/TR/pointerevents3/#the-pointermove-event). Fired when a pointer changes coordinates. Also available via the `on-pointermove` prop.
+- [ ] [`pointerout`](https://www.w3.org/TR/pointerevents3/#the-pointerout-event). Fired when a pointer is moved out of the hit test boundaries of an element (among other reasons). Also available via the `on-pointerout` prop.
+- [ ] [`pointerover`](https://www.w3.org/TR/pointerevents3/#the-pointerover-event). Fired when a pointer is moved into an element's hit test boundaries. Also available via the `on-pointerover` prop.
+- [ ] [`pointerrawupdate`](https://www.w3.org/TR/pointerevents3/#the-pointerrawupdate-event). Fired when a pointer changes any properties that don't fire `pointerdown` or `pointerup` events.
+- [ ] [`pointerup`](https://www.w3.org/TR/pointerevents3/#the-pointerup-event). Fired when a pointer is no longer active. Also available via the `on-pointerup` prop.
+
+## HTMLElement
+
+The HTMLElement interface represents any HTML element. Some elements directly implement this interface, while others implement it via an interface that inherits it.
+
+### Properties
+
+Inherits properties from its parent, Element, and implements those from DocumentAndElementEventHandlers, GlobalEventHandlers, and TouchEventHandlers.
+
+- [ ] [`HTMLElement.hidden`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/hidden) [Read only]. A boolean value indicating if the element is hidden or not.
+- [x] [`HTMLElement.offsetHeight`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/offsetHeight) [Read only]. Returns a double containing the height of an element, relative to the layout.
+- [x] [`HTMLElement.offsetLeft`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/offsetLeft) [Read only]. Returns a double, the distance from this element's left border to its offsetParent's left border.
+- [x] [`HTMLElement.offsetParent`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/offsetParent) [Read only]. Returns a Element that is the element from which all offset calculations are currently computed.
+- [x] [`HTMLElement.offsetTop`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/offsetTop) [Read only]. Returns a double, the distance from this element's top border to its offsetParent's top border.
+- [x] [`HTMLElement.offsetWidth`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/offsetWidth) [Read only]. Returns a double containing the width of an element, relative to the layout.
+
+### Methods
+
+Inherits methods from its parent, Element, and implements those from DocumentAndElementEventHandlers, GlobalEventHandlers, and TouchEventHandlers.
+
+- [x] [`HTMLElement.blur()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/blur). Removes keyboard focus from the currently focused element.
+- [ ] [`HTMLElement.click()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/click). Sends a mouse click event to the element.
+- [ ] [`HTMLElement.focus(options)`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus). Makes the element the current keyboard focus.
+
+### Events
+
+Listen to these events using `addEventListener()` or by assigning an event listener to the equivalent component prop.
+
+- [ ] [`beforeinput`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/beforeinput_event). Fired when the value of an ``, `